In software design, patterns play a crucial role in structuring code, improving maintainability, and enhancing scalability. Abstract Factory Pattern holds significant importance in the world of C# programming. In this article, we’ll delve into the intricacies of the Abstract Factory Pattern, covering its intent, problem-solving capabilities, real-world analogies, implementation structure, applicability, pros and cons, relations with other patterns, step-by-step implementation guide, and code examples.
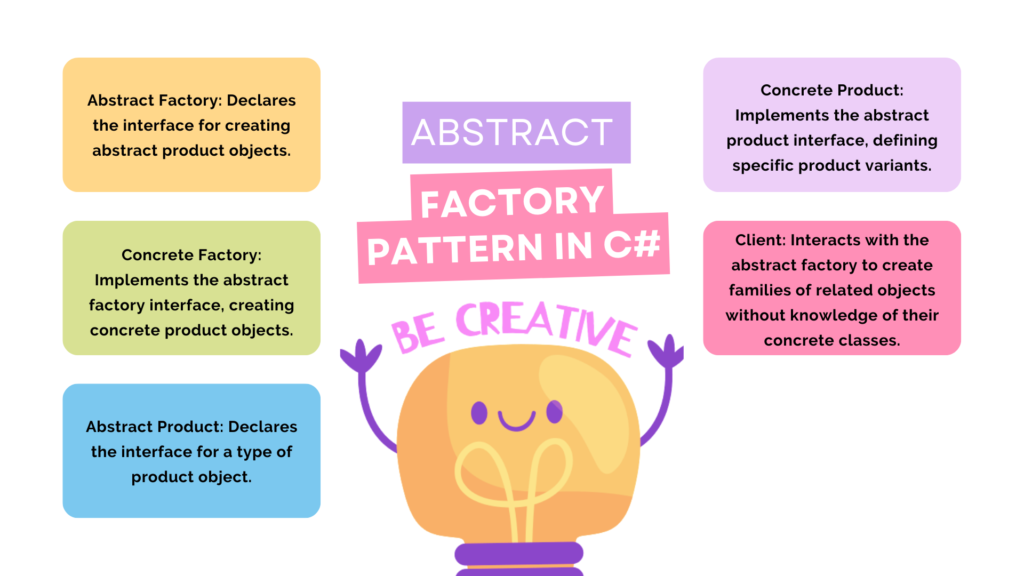
Table of Contents
Intent
The intent of the Abstract Factory Pattern is to provide an interface for creating families of related or dependent objects without specifying their concrete classes. It falls under the creational design patterns category, emphasizing the creation of objects in a manner that is flexible, extensible, and independent of the implementation details.
Problem
When developing applications, you often encounter scenarios where you need to create families of related objects. For example, consider a scenario in an e-commerce application where you need to create different types of products (e.g., electronics, clothing) along with their respective variants (e.g., colors, sizes). Managing the creation of these objects directly in client code can lead to tight coupling, making the codebase rigid and difficult to maintain.
Solution
The Abstract Factory Pattern addresses this problem by defining an abstract factory interface that declares a set of methods for creating each type of object in a family. Concrete implementations of these factories are then provided, each responsible for creating objects of a specific variant within the family. This way, the client code interacts with the abstract factory interface, remaining unaware of the specific classes being instantiated.
Real-World Analogy
Imagine a car manufacturing plant. The plant has different assembly lines for producing various models of cars, such as sedans, SUVs, and electric vehicles. Each assembly line represents a concrete factory that produces components specific to its type of car. The abstract factory, in this context, is the overarching system that defines the interfaces and structures for these assembly lines.
Structure
The key components of the Abstract Factory Pattern include:
- Abstract Factory: Declares the interface for creating abstract product objects.
- Concrete Factory: Implements the abstract factory interface, creating concrete product objects.
- Abstract Product: Declares the interface for a type of product object.
- Concrete Product: Implements the abstract product interface, defining specific product variants.
- Client: Interacts with the abstract factory to create families of related objects without knowledge of their concrete classes.
Applicability
The Abstract Factory Pattern is applicable in scenarios where:
- The system needs to be independent of how its objects are created, composed, and represented.
- The system is configured with multiple families of related objects, and it needs to be able to switch between these families dynamically.
- The creation of object families involves a significant amount of complexity, which can be encapsulated within factory classes.
How to Implement: Abstract Factory Pattern
Let’s go through a step-by-step guide to implementing the Abstract Factory Pattern in C#:
- Define Abstract Factory Interface: Create an interface that declares methods for creating abstract product objects.
public interface IAbstractFactory
{
IAbstractProductA CreateProductA();
IAbstractProductB CreateProductB();
}
- Implement Concrete Factories: Create concrete factory classes that implement the abstract factory interface and provide logic for creating concrete product objects.
public class ConcreteFactory1 : IAbstractFactory
{
public IAbstractProductA CreateProductA()
{
return new ConcreteProductA1();
}
public IAbstractProductB CreateProductB()
{
return new ConcreteProductB1();
}
}
public class ConcreteFactory2 : IAbstractFactory
{
public IAbstractProductA CreateProductA()
{
return new ConcreteProductA2();
}
public IAbstractProductB CreateProductB()
{
return new ConcreteProductB2();
}
}
- Define Abstract Product Interfaces: Create interfaces for abstract product types.
public interface IAbstractProductA
{
void MethodA();
}
public interface IAbstractProductB
{
void MethodB();
}
- Implement Concrete Products: Create concrete product classes that implement the abstract product interfaces.
public class ConcreteProductA1 : IAbstractProductA
{
public void MethodA()
{
Console.WriteLine("Concrete Product A1 - Method A");
}
}
public class ConcreteProductB1 : IAbstractProductB
{
public void MethodB()
{
Console.WriteLine("Concrete Product B1 - Method B");
}
}
// Define ConcreteProductA2, ConcreteProductB2 similarly
- Client Usage: Use the abstract factory to create families of related objects without specifying their concrete classes.
public class Client
{
private readonly IAbstractProductA _productA;
private readonly IAbstractProductB _productB;
public Client(IAbstractFactory factory)
{
_productA = factory.CreateProductA();
_productB = factory.CreateProductB();
}
public void Run()
{
_productA.MethodA();
_productB.MethodB();
}
}
Pros and Cons
Pros:
- Promotes loose coupling between client code and concrete classes.
- Facilitates the creation of families of related objects.
- Supports the addition of new variants of objects without modifying existing client code.
Cons:
- Can lead to a proliferation of interfaces and classes, especially in complex systems.
- Requires careful design to ensure the correct implementation of abstract factories and products.
Relations with Other Patterns
- Factory Method: The Abstract Factory Pattern is often implemented using Factory Methods to create the concrete products within the factory classes.
- Singleton: An Abstract Factory can be a Singleton, ensuring that only one instance of the factory exists throughout the application.
Conclusion
The Abstract Factory Pattern is a powerful tool for managing the creation of families of related objects in a flexible and extensible manner. By encapsulating object creation logic within abstract factories, it promotes code reusability, maintainability, and scalability. When designing applications in C#, especially those with complex object hierarchies and dependencies, the Abstract Factory Pattern proves to be a valuable design choice. Incorporating this pattern into your development can lead to more modular, robust, and maintainable codebases.
Keywords: Abstract Factory Pattern, C#, Creational Design Patterns, Object-Oriented Design, Factory Method, Singleton, Code Examples, Real-World Analogy.
References
For more information and detailed references on the topics discussed in this article, please refer to the following link: