Delegates are a fundamental component of C# programming that play an important role in enabling event handling, callbacks, and dynamic method invocation. This article thoroughly explores definition, functionality, and significance in C# development. It provides clear explanations, insightful examples, and best practices to improve understanding and implementation.
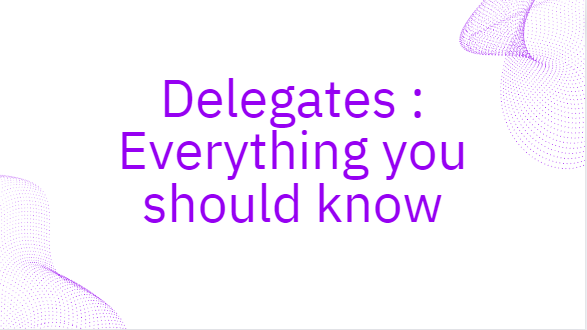
What Are Delegates?
C# delegates are essentially type-safe function pointers that allow us to reference and invoke methods dynamically at runtime. They offer a way to encapsulate and pass methods as inputs, making them extremely adaptable and beneficial in different programming scenarios.
Table of Contents
Role of Delegates in C#:
- Event Handling: These are widely used in event-driven programs to manage events and callbacks. They play the role of connecting event publishers, such as UI controls, with event subscribers, which are methods that respond to events.
- Callback Mechanism: These are essential for implementing callback methods, letting a method be passed as a parameter to another method for dynamic invocation and customizable behavior.
- Decoupling Components: These promote loose coupling between components by enabling one component to call methods on another component without needing to know the exact implementation details.
- Dynamic Invocation: Offer a way to dynamically invoke methods, letting you invoke methods based on runtime conditions or custom logic.
Key Points:
- Encapsulation: Encapsulate methods, providing a clean way to work with them.
- Library Class: Reside in the
System
namespace and are part of the .NET library. - Type-Safe Pointers: They act as type-safe pointers to methods.
- Chaining Methods: You can chain multiple methods to a single delegate, allowing multiple methods to be called when an event occurs.
- Class Agnostic: Don’t care about the class of the object they reference.
- Anonymous Methods and Lambda Expressions: Can be used for invoking “anonymous methods” (C# 2.0) and lambda expressions (C# 3.0).
Steps to Use in C#:
Step 1: Define a Delegate Type
First, you must define the delegate type that defines the signature of the method that the delegate can represent. For example:
delegate void MyDelegate(int x, int y);
Step 2: Create Delegate Instances
Next, instantiate the delegate and link it with methods that match its signature. For instance:
class Program
{
static void Main()
{
MyDelegate delegate1 = new MyDelegate(AddNumbers);
MyDelegate delegate2 = new MyDelegate(SubtractNumbers);
// Invoke
delegate1(5, 3); // Output: 8
delegate2(10, 5); // Output: 5
}
static void AddNumbers(int x, int y)
{
Console.WriteLine($"Sum: {x + y}");
}
static void SubtractNumbers(int x, int y)
{
Console.WriteLine($"Difference: {x - y}");
}
}
Step 3: Invoke
Finally, invoke to execute the linked methods dynamically.
Multicast Delegates
Taking the concept further, another interesting feature of delegates is multicast delegates, which allow you to combine multiple delegate objects into a single delegate instance. Consider the following key points:
- Assigning Multiple Delegates:
- You can assign multiple delegate objects to a delegate instance using the
+
operator. This means that a single delegate instance can point to more than one method.
- You can assign multiple delegate objects to a delegate instance using the
- Combining Delegate Instances:
- Besides using the
+
operator, you can also combine delegate instances using the static methodCombine
. This method creates a new delegate instance that represents the combined invocation of all the delegates it combines.
- Besides using the
- Multicast Delegate:
- A delegate that points to multiple delegates is known as a multicast delegate. This allows you to create a chain of method calls that will be executed in the order they were added to the delegate.
- Invocation List:
- The runtime maintains an invocation list for multicast delegates. This list contains references to all the methods that the delegate will call when invoked.
- Execution Order:
- When a multicast delegate is called, the methods in the invocation list are executed sequentially, following the order in which they were added to the delegate.
Here’s a code snippet example showcasing the usage of the Combine method to form a multicast delegate:
using System;
public class DelegateExample
{
public delegate void MyDelegate();
public static void Method1()
{
Console.WriteLine("Method 1");
}
public static void Method2()
{
Console.WriteLine("Method 2");
}
public static void Main()
{
MyDelegate delegate1 = Method1;
MyDelegate delegate2 = Method2;
// Combine delegate1 and delegate2 into a multicast delegate
MyDelegate multicastDelegate = (MyDelegate)Delegate.Combine(delegate1, delegate2);
// Invoke the multicast delegate
multicastDelegate();
}
}
Here’s a code snippet example showcasing the usage of the += operator to assign the delegate to multiple methods having identical signatures.
public static void Main()
{
MyDelegate delegate1 = Method1;
delegate1 += Method2;
// Invoke the multicast delegate
delegate1.Invoke();
}
Real-Life Applications Examples
Let’s explore more real-life examples to understand their role and practical applications in depth.
Example 1: Event Handling in GUI Applications
Delegates play an important role in handling events in graphical user interface (GUI) applications. Consider a scenario where you have a button in a Windows Forms application, and you want to create a click event for that button
using System;
using System.Windows.Forms;
class Program
{
static void Main()
{
Button button = new Button();
button.Text = "Click Me";
button.Click += new EventHandler(Button_Click);
Form form = new Form();
form.Controls.Add(button);
Application.Run(form);
}
static void Button_Click(object sender, EventArgs e)
{
Console.WriteLine("Button clicked!");
}
}
In this example, the button.Click
event is linked with an EventHandler
delegate, which points to the Button_Click
method. When the button is clicked, the Button_Click
method is invoked, which is how delegates are used to handle events in GUI applications.
Example 2: Asynchronous Programming with Callbacks
Delegation also plays an important role in implementing asynchronous programming techniques such as callbacks. Consider a scenario where you have a method that performs a time-consuming operation asynchronously and notifies the caller when it’s done:
using System;
using System.Threading.Tasks;
class Program
{
delegate Task TaskCompletedCallbackAsync(string result);
static async Task Main()
{
Task task = PerformTaskAsync(async (result) =>
{
Console.WriteLine($"Task completed with result: {result}");
await Task.Yield(); // Ensure asynchronous completion
});
Console.WriteLine("Main method continues executing...");
await task; // Wait for PerformTaskAsync to complete
Console.ReadLine(); // Keep console open
}
static async Task PerformTaskAsync(TaskCompletedCallbackAsync callback)
{
// Simulate async operation
await Task.Delay(3000);
string result = "Task Result";
await callback(result); // Invoke callback when task completes
}
}
In this example, the PerformTaskAsync
method takes a callback delegate (TaskCompletedCallback
) as a parameter calls it back when the asynchronous task is completed. This pattern is commonly used in scenarios like asynchronous file I/O, network operations, and asynchronous APIs.
Example 3: Custom Event Subscription in a Publishing System
To create custom event subscription mechanisms in publishing systems. For instance, consider a publishing system where subscribers can subscribe to different categories of articles:
using System;
using System.Collections.Generic;
class Program
{
delegate void ArticlePublishedEventHandler(string category, string articleTitle);
class Publisher
{
public event ArticlePublishedEventHandler ArticlePublished;
public void PublishArticle(string category, string articleTitle)
{
// Publish the article
Console.WriteLine($"Article '{articleTitle}' published in '{category}' category.");
// Notify subscribers
ArticlePublished?.Invoke(category, articleTitle);
}
}
class Subscriber
{
public void Subscribe(Publisher publisher)
{
publisher.ArticlePublished += HandleArticlePublished;
}
void HandleArticlePublished(string category, string articleTitle)
{
Console.WriteLine($"New article published in '{category}' category: '{articleTitle}'");
}
}
static void Main()
{
Publisher publisher = new Publisher();
Subscriber subscriber1 = new Subscriber();
Subscriber subscriber2 = new Subscriber();
subscriber1.Subscribe(publisher);
subscriber2.Subscribe(publisher);
publisher.PublishArticle("Technology", "Understanding Delegate in C#");
}
}
In this example, the Publisher
class has an ArticlePublished
event of type ArticlePublishedEventHandler
, which is a delegate that points to methods handling article publication events. Subscribers can subscribe to specific categories and receive notifications when new articles are published.
Best Practices for Using Delegates:
- Use Generic Delegates: Prefer generic delegates (
Func
,Action
, etc.) for common delegate scenarios as they provide type-safe and flexible options. - Avoid Circular References: Be mindful of circular references when using delegates to prevent memory leaks and unexpected behavior.
- Handle Null Delegates: Always check for null before invoking delegates to avoid NullReferenceException errors.
- Delegate Combinations: Utilize delegate combination and invocation lists for scenarios requiring multiple method calls through a single delegate instance.
Conclusion:
Delegates are a powerful feature in C# that enable dynamic method invocation, event handling, and callback mechanisms, contributing to the flexibility and extensibility of C# applications. By understanding their role and following best practices, developers can leverage delegates effectively to build robust and modular codebases.
I hope this article clarifies the delegates in C# programming, providing valuable insights and practical examples for developers of all levels. You can refer to Microsoft’s online documentation to learn more about delegates.