In C# programming, data types play an important role in determining the type of data a variable can hold. Understanding basic data types is fundamental for any C# developer. In this article, we’ll delve into the essential data types in C# along with examples to illustrate their usage.
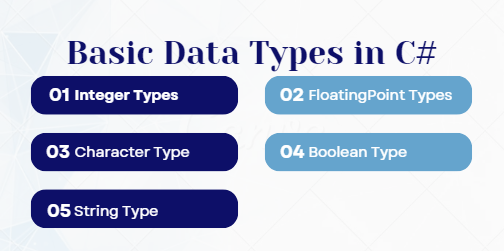
What are Data Types in C#?
Data types in C# define the type and size of data that a variable can store. C# supports various data types, including primitive types and user-defined types.
Basic Data Types in C#
1. Integer Types:
int
: Represents signed 32-bit integers. Example:int age = 30;
uint
: Represents unsigned 32-bit integers. Example:uint count = 100;
short
: Represents signed 16-bit integers. Example:short temperature = -10;
ushort
: Represents unsigned 16-bit integers. Example:ushort quantity = 500;
long
: Represents signed 64-bit integers. Example:long population = 1000000L;
ulong
: Represents unsigned 64-bit integers. Example:ulong distance = 150000UL;
2. Floating-Point Types:
float
: Represents single-precision floating-point numbers. Example:float height = 5.7f;
double
: Represents double-precision floating-point numbers. Example:double pi = 3.14159;
decimal
: Represents decimal numbers with higher precision. Example:decimal price = 99.99m;
3. Character Type:
char
: Represents a single Unicode character. Example:char grade = 'A';
4. Boolean Type:
bool
: Represents a boolean value (true or false). Example:bool isStudent = true;
5. String Type:
string
: Represents a sequence of characters. Example:string name = "John Doe";
Examples and Usage:
using System;
class Program
{
static void Main()
{
// Integer Types
int age = 30;
uint count = 100;
short temperature = -10;
ushort quantity = 500;
long population = 1000000L;
ulong distance = 150000UL;
// Floating-Point Types
float height = 5.7f;
double pi = 3.14159;
decimal price = 99.99m;
// Character Type
char grade = 'A';
// Boolean Type
bool isStudent = true;
// String Type
string name = "John Doe";
Console.WriteLine("Age: " + age);
Console.WriteLine("Count: " + count);
Console.WriteLine("Temperature: " + temperature);
Console.WriteLine("Quantity: " + quantity);
Console.WriteLine("Population: " + population);
Console.WriteLine("Distance: " + distance);
Console.WriteLine("Height: " + height);
Console.WriteLine("Pi: " + pi);
Console.WriteLine("Price: " + price);
Console.WriteLine("Grade: " + grade);
Console.WriteLine("Is Student: " + isStudent);
Console.WriteLine("Name: " + name);
}
}
In this example, we’ve declared variables of various basic data types and assigned values to them. The Main
method then prints out the values of these variables to the console.
Conclusion:
Understanding basic data types is foundational in C# programming, as it allows developers to work with different kinds of data efficiently. By mastering these data types, developers can write robust and reliable C# code.
I hope this article helps you grasp the basics of data types in C# programming. Happy coding!